OOP第二次作业总结(222019班22201321)
一、前言
这次作业总结主要是总结分析训练集04的7-1、训练集05的7-5和7-6、训练集06的7-4和7-5。
三次题目涉及的的知识点主要有:面向对象的封装、聚合、继承,还练习了正则表达式的使用以及字符串的处理等等。
难度对我来说,非常大,这三次训练集相比上一次难度提升了简直不是一个度,不熬夜加班写根本写不完,虽然我熬的夜还是没能让我写完某些题目。一些设计需求简单的、只是单纯练习语法的题目还好,对于那几道设计较为复杂,需求多且复杂的题目,我的确很难下手,训练集04和05的设计类题目给了类图会容易着手一点,训练集06的7-4和7-5没给设计类图让我一下不知所措了,这需要自行设计,需要一个良好的逻辑和设计思维,训练到此,我可能还未达到标准,因此这最后一次的题目对我来说非常困难。
二、设计与分析
1.日期问题面向对象设计(聚合一)
设计如下几个类:DateUtil、Year、Month、Day,其中年、月、日的取值范围依然为:year∈[1900,2050] ,month∈[1,12] ,day∈[1,31] , 设计类图如下:
这道题与之前设计的日期类相似,只是要求以聚合的方式重新设计。
按照题目提供的类图,顺延其思路设计下去就行。
以下是我的代码:

1 import java.util.*; 2 3 public class Main { 4 public static void main(String[] args) { 5 Scanner input = new Scanner(System.in); 6 int year = 0; 7 int month = 0; 8 int day = 0; 9 10 int choice = input.nextInt(); 11 12 if (choice == 1) { // test getNextNDays method 13 int m = 0; 14 year = input.nextInt(); 15 month = input.nextInt(); 16 day = input.nextInt(); 17 m = input.nextInt(); 18 19 DateUtil date = new DateUtil(year, month, day); 20 21 if (!date.checkInputValidity()) { 22 System.out.println("Wrong Format"); 23 System.exit(0); 24 } 25 26 if (m < 0) { 27 System.out.println("Wrong Format"); 28 System.exit(0); 29 } 30 31 System.out.println(date.getNextNDays(m).showDate()); 32 } 33 else if (choice == 2) { // test getPreviousNDays method 34 int n = 0; 35 year = input.nextInt(); 36 month = input.nextInt(); 37 day = input.nextInt(); 38 n = input.nextInt(); 39 DateUtil date = new DateUtil(year, month, day); 40 41 if (!date.checkInputValidity()) { 42 System.out.println("Wrong Format"); 43 System.exit(0); 44 } 45 46 if (n < 0) { 47 System.out.println("Wrong Format"); 48 System.exit(0); 49 } 50 51 System.out.println(date.getPreviousNDays(n).showDate()); 52 } 53 else if (choice == 3) { //test getDaysofDates method 54 year = input.nextInt(); 55 month = input.nextInt(); 56 day = input.nextInt(); 57 58 int anotherYear = input.nextInt(); 59 int anotherMonth = input.nextInt(); 60 int anotherDay = input.nextInt(); 61 62 DateUtil fromDate = new DateUtil(year, month, day); 63 DateUtil toDate = new DateUtil(anotherYear, anotherMonth, anotherDay); 64 65 if (fromDate.checkInputValidity() && toDate.checkInputValidity()) { 66 System.out.println(fromDate.getDaysofDates(toDate)); 67 } 68 else { 69 System.out.println("Wrong Format"); 70 System.exit(0); 71 } 72 } 73 else{ 74 System.out.println("Wrong Format"); 75 System.exit(0); 76 } 77 } 78 static class Day { 79 private int value; 80 public int getValue() { 81 return value; 82 } 83 public void setValue(int value) { 84 this.value = value; 85 } 86 private Month month=new Month(); 87 int[] mon_maxnum={31,28,31,30,31,30,31,31,30,31,30,31}; 88 89 public Month getMonth() { 90 return month; 91 } 92 public void setMonth(Month month) { 93 this.month = month; 94 } 95 public Day(){} 96 public Day(int yearValue,int monthValue,int dayValue){ 97 this.month=new Month(yearValue,monthValue); 98 this.value=dayValue; 99 } 100 public void resetMin(){ 101 this.value=1; 102 } 103 public void resetMax(){ 104 if(this.getMonth().getYear().isLeapYear()){ 105 mon_maxnum[1]=29; 106 } 107 108 else { 109 mon_maxnum[1]=28; 110 } 111 this.value=mon_maxnum[this.month.getValue()-1]; 112 } 113 public void dayIncreament(){ 114 this.value+=1; 115 } 116 public void dayReduction(){ 117 this.value-=1; 118 } 119 public boolean validate(){ 120 if (this.month.getYear().isLeapYear()) { 121 mon_maxnum[1] = 29; 122 } 123 else { 124 mon_maxnum[1] = 28; 125 } 126 if (this.value >= 1 && this.value <= mon_maxnum[this.month.getValue() - 1]) 127 return true; 128 else 129 return false; 130 } 131 } 132 static class Month { 133 private int value; 134 public int getValue() { 135 return value; 136 } 137 public void setValue(int value) { 138 this.value = value; 139 } 140 141 private Year year= new Year(); 142 public Year getYear() { 143 return year; 144 } 145 public void setYear(Year year) { 146 this.year = year; 147 } 148 149 public Month(){} 150 public Month(int yearValue,int monthValue){ 151 this.value=monthValue; 152 this.year=new Year(yearValue); 153 } 154 155 public void resetMin(){ 156 this.value=1; 157 } 158 public void resetMax(){ 159 this.value=12; 160 } 161 public boolean validate(){ 162 if(this.value>=1&&this.value<=12) 163 return true; 164 else 165 return false; 166 } 167 public void monthIncrement(){//月份加一 168 this.value+=1; 169 } 170 public void monthReduction(){//月份减一 171 this.value-=1; 172 } 173 } 174 static class Year { 175 private int value; 176 public int getValue() { 177 return value; 178 } 179 public void setValue(int value) { 180 this.value = value; 181 } 182 public Year(){} 183 public Year(int value){ 184 this.value = value; 185 } 186 public boolean isLeapYear() {//判断year是否为闰年 187 if (this.value % 4 == 0 && this.value % 100 != 0 || this.value % 400 == 0) 188 return true; 189 else 190 return false; 191 } 192 public boolean validate(){ 193 if(this.value>=1900&&this.value<=2050) 194 return true; 195 else 196 return false; 197 } 198 public void yearIncrement(){ 199 this.value+=1; 200 } 201 public void yearReduction(){ 202 this.value-=1; 203 } 204 } 205 static class DateUtil { 206 private Day day; 207 208 public Day getDay() { 209 return day; 210 } 211 212 public void setDay(Day day) { 213 this.day = day; 214 } 215 216 public DateUtil() {} 217 218 public DateUtil(int y, int m, int d) { 219 this.day = new Day(y, m, d); 220 } 221 222 public boolean checkInputValidity() {//校验数据合法性 223 if (this.day.getMonth().validate() && this.day.getMonth().getYear().validate()&&this.day.validate()) { 224 return true; 225 } 226 else { 227 return false; 228 } 229 } 230 231 public boolean compareDates(DateUtil date) {//比较两个日期大小 232 if (this.day.getMonth().getYear().getValue() > date.day.getMonth().getYear().getValue()) { 233 return true; 234 } 235 else if (this.day.getMonth().getYear().getValue() < date.day.getMonth().getYear().getValue()) { 236 return false; 237 } 238 else { 239 if (this.day.getMonth().getValue() > date.day.getMonth().getValue()) { 240 return true; 241 } 242 else if (this.day.getMonth().getValue() < date.day.getMonth().getValue()) { 243 return false; 244 } 245 else { 246 if (this.day.getValue() > date.day.getValue()) { 247 return true; 248 } 249 else { 250 return false; 251 } 252 } 253 } 254 } 255 256 public boolean equalTwoDates(DateUtil date) {//判断两个日期是否相等 257 if (this.day.getValue() == date.day.getValue() && 258 this.day.getMonth().getValue() == date.day.getMonth().getValue() && 259 this.day.getMonth().getYear().getValue() == date.day.getMonth().getYear().getValue()) { 260 return true; 261 } 262 else { 263 return false; 264 } 265 } 266 267 public String showDate() {//日期值格式化 268 return this.day.getMonth().getYear().getValue() + "-" + 269 this.day.getMonth().getValue() + "-" + this.day.getValue(); 270 } 271 272 public DateUtil getNextNDays(int n) {//求下n天 273 DateUtil nextDate = new DateUtil(this.day.getMonth().getYear().getValue(), 274 this.day.getMonth().getValue(), this.day.getValue()); 275 for (int i = 0; i < n; i++) { 276 nextDate.day.dayIncreament(); 277 if (!nextDate.day.validate()) { 278 nextDate.day.resetMin(); 279 nextDate.day.getMonth().monthIncrement(); 280 if (!nextDate.day.getMonth().validate()) { 281 nextDate.day.getMonth().resetMin(); 282 nextDate.day.getMonth().getYear().yearIncrement(); 283 } 284 } 285 } 286 return nextDate; 287 } 288 289 public DateUtil getPreviousNDays(int n) {//求前n天 290 DateUtil prevDate = new DateUtil(this.day.getMonth().getYear().getValue(), 291 this.day.getMonth().getValue(), this.day.getValue()); 292 for (int i = 0; i < n; i++) { 293 prevDate.day.dayReduction(); 294 if (!prevDate.day.validate()) { 295 296 prevDate.day.getMonth().monthReduction(); 297 if (!prevDate.day.getMonth().validate()) { 298 prevDate.day.getMonth().resetMax(); 299 prevDate.day.getMonth().getYear().yearReduction(); 300 } 301 prevDate.day.resetMax(); 302 } 303 } 304 return prevDate; 305 } 306 307 public int getDaysofDates(DateUtil date) {//求两个日期之间的天数 308 int days = 0; 309 DateUtil startDate = new DateUtil(); 310 DateUtil endDate = new DateUtil(); 311 if (this.compareDates(date)) { 312 startDate = date; 313 endDate = this; 314 } 315 else { 316 startDate = this; 317 endDate = date; 318 } 319 while (!startDate.equalTwoDates(endDate)) { 320 startDate = startDate.getNextNDays(1); 321 days += 1; 322 } 323 return days; 324 } 325 } 326 }
这道题因为之前也做过几道日期类设计的题目,没有做过多思考,更多的是直接贴合需求去解题。
2.日期问题面向对象设计(聚合二)
设计如下几个类:DateUtil、Year、Month、Day,其中年、月、日的取值范围依然为:year∈[1820,2020] ,month∈[1,12] ,day∈[1,31] , 设计类图如下:
以下为我的代码:

1 import java.util.Scanner; 2 3 public class Main { 4 public static void main(String[] args){ 5 Scanner input = new Scanner(System.in); 6 int year = 0; 7 int month = 0; 8 int day = 0; 9 10 int choice = input.nextInt(); 11 12 if (choice == 1) { // test getNextNDays method 13 int m = 0; 14 year = input.nextInt(); 15 month = input.nextInt(); 16 day = input.nextInt(); 17 m = input.nextInt(); 18 19 DateUtil date = new DateUtil(year, month, day); 20 21 if (!date.checkInputValidity()) { 22 System.out.println("Wrong Format"); 23 System.exit(0); 24 } 25 26 if (m < 0) { 27 System.out.println("Wrong Format"); 28 System.exit(0); 29 } 30 System.out.print(date.getYear().getValue() + "-" + date.getMonth().getValue() + "-" + date.getDay().getValue() + " next " + m + " days is:"); 31 System.out.println(date.getNextNDays(m).showDate()); 32 } 33 else if (choice == 2) { // test getPreviousNDays method 34 int n = 0; 35 year = input.nextInt(); 36 month = input.nextInt(); 37 day = input.nextInt(); 38 n = input.nextInt(); 39 DateUtil date = new DateUtil(year, month, day); 40 41 if (!date.checkInputValidity()) { 42 System.out.println("Wrong Format"); 43 System.exit(0); 44 } 45 46 if (n < 0) { 47 System.out.println("Wrong Format"); 48 System.exit(0); 49 } 50 System.out.print( 51 date.getYear().getValue() + "-" + date.getMonth().getValue() + "-" + date.getDay().getValue() + " previous " + n + " days is:"); 52 53 System.out.println(date.getPreviousNDays(n).showDate()); 54 } 55 else if (choice == 3) { //test getDaysofDates method 56 year = input.nextInt(); 57 month = input.nextInt(); 58 day = input.nextInt(); 59 60 int anotherYear = input.nextInt(); 61 int anotherMonth = input.nextInt(); 62 int anotherDay = input.nextInt(); 63 64 DateUtil fromDate = new DateUtil(year, month, day); 65 DateUtil toDate = new DateUtil(anotherYear, anotherMonth, anotherDay); 66 67 if (fromDate.checkInputValidity() && toDate.checkInputValidity()) { 68 System.out.print("The days between " + fromDate.showDate() + 69 " and " + toDate.showDate() + " are:"); 70 System.out.println(fromDate.getDaysofDates(toDate)); 71 } 72 else { 73 System.out.println("Wrong Format"); 74 System.exit(0); 75 } 76 } 77 else{ 78 System.out.println("Wrong Format"); 79 System.exit(0); 80 } 81 } 82 static class Year { 83 private int value; 84 public int getValue() { 85 return value; 86 } 87 public void setValue(int value) { 88 this.value = value; 89 } 90 public Year(){} 91 public Year(int value){ 92 this.value = value; 93 } 94 public boolean isLeapYear() {//判断year是否为闰年 95 if ((this.value % 4 == 0 && this.value % 100 != 0) || this.value % 400 == 0) 96 return true; 97 else 98 return false; 99 } 100 public boolean validate(){ 101 if(this.value>=1820&&this.value<=2020) 102 return true; 103 else 104 return false; 105 } 106 public void yearIncrement(){ 107 this.value+=1; 108 } 109 public void yearReduction(){ 110 this.value-=1; 111 } 112 } 113 static class Month { 114 private int value; 115 public int getValue() { 116 return value; 117 } 118 public void setValue(int value) { 119 this.value = value; 120 } 121 122 public Month(){} 123 public Month(int Value){ 124 this.value=Value; 125 } 126 127 public void resetMin(){ 128 this.value=1; 129 } 130 public void resetMax(){ 131 this.value=12; 132 } 133 public boolean validate(){ 134 if(this.value>=1&&this.value<=12) 135 return true; 136 else 137 return false; 138 } 139 public void monthIncrement(){//月份加一 140 this.value+=1; 141 } 142 public void monthReduction(){//月份减一 143 this.value-=1; 144 } 145 } 146 static class Day { 147 private int value; 148 public int getValue() { 149 return value; 150 } 151 public void setValue(int value) { 152 this.value = value; 153 } 154 155 public Day(){} 156 public Day(int Value){ 157 this.value=Value; 158 } 159 160 public void dayIncreament(){ 161 this.value+=1; 162 } 163 public void dayReduction(){ 164 this.value-=1; 165 } 166 167 } 168 static class DateUtil { 169 private Day day; 170 private Month month; 171 private Year year; 172 int[] mon_maxnum={31,28,31,30,31,30,31,31,30,31,30,31}; 173 174 public Day getDay() { 175 return day; 176 } 177 178 public void setDay(Day day) { 179 this.day = day; 180 } 181 182 public Month getMonth() { 183 return month; 184 } 185 186 public void setMonth(Month month) { 187 this.month = month; 188 } 189 190 public Year getYear() { 191 return year; 192 } 193 194 public void setYear(Year year) { 195 this.year = year; 196 } 197 198 public DateUtil() {} 199 200 public DateUtil(int y, int m, int d) { 201 this.year=new Year(y); 202 this.month=new Month(m); 203 this.day=new Day(d); 204 } 205 public void setDayMin(){ 206 this.day.setValue(1); 207 } 208 public void setDayMax(){ 209 if(this.year.isLeapYear()){ 210 mon_maxnum[1]=29; 211 } 212 else { 213 mon_maxnum[1]=28; 214 } 215 this.day.setValue(mon_maxnum[this.month.getValue()-1]); 216 } 217 218 public boolean checkInputValidity() {//校验数据合法性 219 if(this.year.isLeapYear()){ 220 mon_maxnum[1]=29; 221 } 222 else { 223 mon_maxnum[1]=28; 224 } 225 if (this.month.validate() && this.year.validate()&&this.day.getValue() >= 1 && this.day.getValue() <= mon_maxnum[this.month.getValue() - 1]) { 226 return true; 227 } 228 else { 229 return false; 230 } 231 } 232 233 public boolean compareDates(DateUtil date) {//比较两个日期大小 234 if (this.year.getValue() > date.year.getValue()) { 235 return true; 236 } 237 else if (this.year.getValue() < date.year.getValue()) { 238 return false; 239 } 240 else { 241 if (this.month.getValue() > date.month.getValue()) { 242 return true; 243 } 244 else if (this.month.getValue() < date.month.getValue()) { 245 return false; 246 } 247 else { 248 if (this.day.getValue() > date.day.getValue()) { 249 return true; 250 } 251 else { 252 return false; 253 } 254 } 255 } 256 } 257 258 public boolean equalTwoDates(DateUtil date) {//判断两个日期是否相等 259 if (this.day.getValue() == date.day.getValue() && 260 this.month.getValue() == date.month.getValue() && 261 this.year.getValue() == date.year.getValue()) { 262 return true; 263 } 264 else { 265 return false; 266 } 267 } 268 269 public String showDate() {//日期值格式化 270 return this.year.getValue() + "-" + 271 this.month.getValue() + "-" + this.day.getValue(); 272 } 273 274 public DateUtil getNextNDays(int n) {//求下n天 275 DateUtil nextDate = new DateUtil(this.year.getValue(), 276 this.month.getValue(), this.day.getValue()); 277 for (int i = 0; i < n; i++) { 278 if(nextDate.year.isLeapYear()) { 279 mon_maxnum[1] = 29; 280 } 281 else { 282 mon_maxnum[1] = 28; 283 } 284 if(nextDate.month.getValue()==12&&nextDate.day.getValue()==31){ 285 nextDate.year.yearIncrement(); 286 nextDate.month.resetMin(); 287 nextDate.setDayMin(); 288 } 289 else if(nextDate.month.getValue()!=12 && nextDate.day.getValue()==mon_maxnum[nextDate.month.getValue()-1]){ 290 nextDate.month.monthIncrement(); 291 nextDate.setDayMin(); 292 } 293 else{ 294 nextDate.day.dayIncreament(); 295 } 296 } 297 return nextDate; 298 } 299 300 public DateUtil getPreviousNDays(int n) {//求前n天 301 DateUtil prevDate = new DateUtil(this.year.getValue(), 302 this.month.getValue(), this.day.getValue()); 303 for (int i = 0; i < n; i++) { 304 prevDate.day.dayReduction(); 305 if (prevDate.day.getValue()<1||prevDate.day.getValue()>mon_maxnum[prevDate.month.getValue()-1]) { 306 307 prevDate.month.monthReduction(); 308 if (!prevDate.month.validate()) { 309 prevDate.month.resetMax(); 310 prevDate.year.yearReduction(); 311 } 312 prevDate.setDayMax(); 313 } 314 } 315 return prevDate; 316 } 317 318 public int getDaysofDates(DateUtil date) {//求两个日期之间的天数 319 int days = 0; 320 DateUtil startDate = new DateUtil(); 321 DateUtil endDate = new DateUtil(); 322 if (this.compareDates(date)) { 323 startDate = date; 324 endDate = this; 325 } 326 else { 327 startDate = this; 328 endDate = date; 329 } 330 while (!startDate.equalTwoDates(endDate)) { 331 startDate = startDate.getNextNDays(1); 332 days += 1; 333 } 334 return days; 335 } 336 } 337 338 }
这道题在7-5的基础上写,比较顺利,主要就是进行一个解耦,之前7-5代码中年、月、日三个类之间的耦合度较强。
3.菜单计价程序-3
输入内容按先后顺序包括两部分:菜单、订单,最后以"end"结束。
菜单由一条或多条菜品记录组成,每条记录一行
每条菜品记录包含:菜名、基础价格 两个信息。
订单分:桌号标识、点菜记录和删除信息、代点菜信息。每一类信息都可包含一条或多条记录,每条记录一行或多行。
桌号标识独占一行,包含两个信息:桌号、时间。
桌号以下的所有记录都是本桌的记录,直至下一个桌号标识。
点菜记录包含:序号、菜名、份额、份数。份额可选项包括:1、2、3,分别代表小、中、大份。
不同份额菜价的计算方法:小份菜的价格=菜品的基础价格。中份菜的价格=菜品的基础价格1.5。小份菜的价格=菜品的基础价格2。如果计算出现小数,按四舍五入的规则进行处理。
删除记录格式:序号 delete
标识删除对应序号的那条点菜记录。
如果序号不对,输出"delete error"
代点菜信息包含:桌号 序号 菜品名称 份额 分数
代点菜是当前桌为另外一桌点菜,信息中的桌号是另一桌的桌号,带点菜的价格计算在当前这一桌。
程序最后按输入的先后顺序依次输出每一桌的总价(注意:由于有代点菜的功能,总价不一定等于当前桌上的菜的价格之和)。
每桌的总价等于那一桌所有菜的价格之和乘以折扣。如存在小数,按四舍五入规则计算,保留整数。
折扣的计算方法(注:以下时间段均按闭区间计算):
周一至周五营业时间与折扣:晚上(17:00-20:30)8折,周一至周五中午(10:30--14:30)6折,其余时间不营业。
周末全价,营业时间:9:30-21:30
如果下单时间不在营业范围内,输出"table " + t.tableNum + " out of opening hours"

参考以下类的模板进行设计:菜品类:对应菜谱上一道菜的信息。 Dish { String name;//菜品名称 int unit_price; //单价 int getPrice(int portion)//计算菜品价格的方法,输入参数是点菜的份额(输入数据只能是1/2/3,代表小/中/大份) } 菜谱类:对应菜谱,包含饭店提供的所有菜的信息。 Menu { Dish\[\] dishs ;//菜品数组,保存所有菜品信息 Dish searthDish(String dishName)//根据菜名在菜谱中查找菜品信息,返回Dish对象。 Dish addDish(String dishName,int unit_price)//添加一道菜品信息 } 点菜记录类:保存订单上的一道菜品记录 Record { int orderNum;//序号\\ Dish d;//菜品\\ int portion;//份额(1/2/3代表小/中/大份)\\ int getPrice()//计价,计算本条记录的价格\\ } 订单类:保存用户点的所有菜的信息。 Order { Record\[\] records;//保存订单上每一道的记录 int getTotalPrice()//计算订单的总价 Record addARecord(int orderNum,String dishName,int portion,int num)//添加一条菜品信息到订单中。 delARecordByOrderNum(int orderNum)//根据序号删除一条记录 findRecordByNum(int orderNum)//根据序号查找一条记录 } ### 输入格式: 桌号标识格式:table + 序号 +英文空格+ 日期(格式:YYYY/MM/DD)+英文空格+ 时间(24小时制格式: HH/MM/SS) 菜品记录格式: 菜名+英文空格+基础价格 如果有多条相同的菜名的记录,菜品的基础价格以最后一条记录为准。 点菜记录格式:序号+英文空格+菜名+英文空格+份额+英文空格+份数注:份额可输入(1/2/3), 1代表小份,2代表中份,3代表大份。 删除记录格式:序号 +英文空格+delete 代点菜信息包含:桌号+英文空格+序号+英文空格+菜品名称+英文空格+份额+英文空格+分数 最后一条记录以“end”结束。 ### 输出格式: 按输入顺序输出每一桌的订单记录处理信息,包括: 1、桌号,格式:table+英文空格+桌号+”:” 2、按顺序输出当前这一桌每条订单记录的处理信息, 每条点菜记录输出:序号+英文空格+菜名+英文空格+价格。其中的价格等于对应记录的菜品\*份数,序号是之前输入的订单记录的序号。如果订单中包含不能识别的菜名,则输出“\*\* does not exist”,\*\*是不能识别的菜名 如果删除记录的序号不存在,则输出“delete error” 最后按输入顺序一次输出每一桌所有菜品的总价(整数数值)格式:table+英文空格+桌号+“:”+英文空格+当前桌的总价 本次题目不考虑其他错误情况,如:桌号、菜单订单顺序颠倒、不符合格式的输入、序号重复等,在本系列的后续作业中会做要求。 输入格式: 桌号标识格式:table + 序号 +英文空格+ 日期(格式:YYYY/MM/DD)+英文空格+ 时间(24小时制格式: HH/MM/SS) 菜品记录格式: 菜名+英文空格+基础价格 如果有多条相同的菜名的记录,菜品的基础价格以最后一条记录为准。 点菜记录格式:序号+英文空格+菜名+英文空格+份额+英文空格+份数注:份额可输入(1/2/3), 1代表小份,2代表中份,3代表大份。 删除记录格式:序号 +英文空格+delete 代点菜信息包含:桌号+英文空格+序号+英文空格+菜品名称+英文空格+份额+英文空格+分数 最后一条记录以“end”结束。 输出格式: 按输入顺序输出每一桌的订单记录处理信息,包括: 1、桌号,格式:table+英文空格+桌号+“:” 2、按顺序输出当前这一桌每条订单记录的处理信息, 每条点菜记录输出:序号+英文空格+菜名+英文空格+价格。其中的价格等于对应记录的菜品\*份数,序号是之前输入的订单记录的序号。如果订单中包含不能识别的菜名,则输出“\*\* does not exist”,\*\*是不能识别的菜名 如果删除记录的序号不存在,则输出“delete error” 最后按输入顺序一次输出每一桌所有菜品的总价(整数数值)格式:table+英文空格+桌号+“:”+英文空格+当前桌的总价 本次题目不考虑其他错误情况,如:桌号、菜单订单顺序颠倒、不符合格式的输入、序号重复等,在本系列的后续作业中会做要求。
这道题难度对我来说极高,我并没有在规定时间内写出这道题。
如下为我设计的类图(尚不完善):
这道题我尚还在钻研中,对我来说难度太大了,还须大量改进。
4.ATM机类结构设计(一)
题目说明:https://images.ptausercontent.com/93fc7ad6-5e85-445a-a759-5790d0baab28.pdf
以下为我的代码:

1 import java.util.ArrayList; 2 import java.util.Scanner; 3 4 public class Main { 5 6 public static void main(String[] args) { 7 ChinaUnionPay chinaUnionPay=init(); // 初始化数据 8 Scanner scanner = new Scanner(System.in); 9 String line; 10 StringBuilder sb=new StringBuilder(); 11 line=scanner.nextLine(); 12 while (!line.equals("#")) { 13 sb.append(line + "\n"); 14 line = scanner.nextLine(); 15 } 16 Deal deal=new Deal(chinaUnionPay,sb); 17 deal.getDealResult(); 18 19 } 20 21 // 初始化数据 22 private static ChinaUnionPay init() { 23 Card card1=new Card("6217000010041315709"); 24 Card card2=new Card("6217000010041315715"); 25 Card card3=new Card("6217000010041315718"); 26 Card card4=new Card("6217000010051320007"); 27 Card card5=new Card("6222081502001312389"); 28 Card card6=new Card("6222081502001312390"); 29 Card card7=new Card("6222081502001312399"); 30 Card card8=new Card("6222081502001312400"); 31 Card card9=new Card("6222081502051320785"); 32 Card card10=new Card("6222081502051320786"); 33 Card[] cards1={card1,card2}; 34 Card[] cards2={card3}; 35 Card[] cards3={card4}; 36 Card[] cards4={card5}; 37 Card[] cards5={card6}; 38 Card[] cards6={card7,card8}; 39 Card[] cards7={card9}; 40 Card[] cards8={card10}; 41 42 Account account1=new Account("3217000010041315709",cards1); 43 Account account2=new Account("3217000010041315715",cards2); 44 Account account3=new Account("3217000010051320007",cards3); 45 Account account4=new Account("3222081502001312389",cards4); 46 Account account5=new Account("3222081502001312390",cards5); 47 Account account6=new Account("3222081502001312399",cards6); 48 Account account7=new Account("3222081502051320785",cards7); 49 Account account8=new Account("3222081502051320786",cards8); 50 Account[] accounts1={account1,account2}; 51 Account[] accounts2={account3}; 52 Account[] accounts3={account4,account5,account6}; 53 Account[] accounts4={account7,account8}; 54 55 ATM atm1=new ATM("01"); 56 ATM atm2=new ATM("02"); 57 ATM atm3=new ATM("03"); 58 ATM atm4=new ATM("04"); 59 ATM atm5=new ATM("05"); 60 ATM atm6=new ATM("06"); 61 ATM[] atms1={atm1,atm2,atm3,atm4}; 62 ATM[] atms2={atm5,atm6}; 63 64 65 User user1=new User("杨过",accounts1); 66 User user2=new User("郭靖",accounts2); 67 User user3=new User("张无忌",accounts3); 68 User user4=new User("韦小宝",accounts4); 69 User[] users1={user1,user2}; 70 User[] users2={user3,user4}; 71 72 Bank bank1=new Bank("中国建设银行",atms1,users1); 73 Bank bank2=new Bank("中国工商银行",atms2,users2); 74 Bank[] banks={bank1,bank2}; 75 76 77 ChinaUnionPay chinaUnionPay=new ChinaUnionPay(banks); 78 return chinaUnionPay; 79 } 80 81 public static class User { 82 private String userName; 83 private Account[] accounts; 84 public User(){} 85 public User(String userName,Account[] accounts){ 86 this.userName=userName; 87 this.accounts=accounts; 88 } 89 90 public Account[] getAccounts() { 91 return accounts; 92 } 93 94 public void setAccounts(Account[] accounts) { 95 this.accounts = accounts; 96 } 97 98 public String getUserName() { 99 return userName; 100 } 101 102 public void setUserName(String userName) { 103 this.userName = userName; 104 } 105 } 106 107 public static class Deal { 108 private ChinaUnionPay chinaUnionPay; 109 private StringBuilder sb; 110 public Deal(){} 111 public Deal(ChinaUnionPay chinaUnionPay,StringBuilder sb){ 112 this.chinaUnionPay=chinaUnionPay; 113 this.sb=sb; 114 } 115 116 public StringBuilder getSb() { 117 return sb; 118 } 119 120 public void setSb(StringBuilder sb) { 121 this.sb = sb; 122 } 123 124 public ChinaUnionPay getChinaUnionPay() { 125 return chinaUnionPay; 126 } 127 128 public void setChinaUnionPay(ChinaUnionPay chinaUnionPay) { 129 this.chinaUnionPay = chinaUnionPay; 130 } 131 public void getDealResult(){ 132 String datas= sb.toString(); 133 String[] data=datas.split("\n"); 134 for(int i=0;i<data.length;i++){ 135 CheckData checkData=new CheckData(data[i],this.chinaUnionPay); 136 if(checkData.validateData()){ 137 String part[] =data[i].split(" "); 138 ArrayList<String> arrayList=new ArrayList<>(); 139 for (int j=0;j< part.length;j++){ 140 arrayList.add(part[j]); 141 arrayList.remove(""); 142 } 143 if(arrayList.size()==4){ 144 CheckCard checkCard1=new CheckCard(this.chinaUnionPay,arrayList.get(2)); 145 changeBalance(checkCard1,arrayList.get(0), Double.parseDouble(arrayList.get(3))); 146 } 147 else if(arrayList.size()==1){ 148 CheckCard checkCard2=new CheckCard(this.chinaUnionPay); 149 Account account=checkCard2.QueryAccount(arrayList.get(0)); 150 System.out.print("¥"); 151 System.out.printf("%.2f\n",account.getBalance()); 152 } 153 } 154 else if(!checkData.validateData()){ 155 return; 156 } 157 } 158 } 159 public void changeBalance(CheckCard checkCard,String cardNumber, double money) { //存钱或取钱 160 Account account = checkCard.QueryAccount(cardNumber); 161 User user = checkCard.QueryUser(cardNumber); 162 Bank bank = checkCard.QueryBank(cardNumber); 163 account.setBalance(account.getBalance() - money); 164 if (money > 0) { 165 System.out.println(user.getUserName() + "在" + bank.getName() + "的" + checkCard.getATMid() + "号ATM机上取款¥" + String.format("%.2f", money) + "\n" + "当前余额为¥" + String.format("%.2f", account.getBalance())); 166 } else { 167 System.out.println(user.getUserName() + "在" + bank.getName() + "的" + checkCard.getATMid() + "号ATM机上存款¥" + String.format("%.2f", -money) + "\n" + "当前余额为¥" + String.format("%.2f", account.getBalance())); 168 } 169 } 170 } 171 172 public static class ChinaUnionPay { 173 private Bank[] banks; 174 public ChinaUnionPay(){} 175 public ChinaUnionPay(Bank[] banks){ 176 this.banks=banks; 177 } 178 179 public Bank[] getBanks() { 180 return banks; 181 } 182 183 public void setBanks(Bank[] banks) { 184 this.banks = banks; 185 } 186 187 } 188 189 public static class CheckData { 190 private String datas; 191 private ChinaUnionPay chinaUnionPay; 192 193 public CheckData() { 194 } 195 public CheckData(String data, ChinaUnionPay chinaUnionPay){ 196 this.chinaUnionPay = chinaUnionPay; 197 this.datas = data; 198 } 199 200 public ChinaUnionPay getChinaUnionPay() { 201 return chinaUnionPay; 202 } 203 204 public void setChinaUnionPay(ChinaUnionPay chinaUnionPay) { 205 this.chinaUnionPay = chinaUnionPay; 206 } 207 208 public String getData() { 209 return datas; 210 } 211 212 public void setData(String datas) { 213 this.datas = datas; 214 } 215 public boolean validateGetMoney(double withdrawMoney, Account account) { 216 if (withdrawMoney > account.getBalance()) { 217 return false; 218 } 219 else 220 return true; 221 } 222 public boolean validatePassword(String password){ 223 if(password.matches("88888888")){ 224 return true; 225 } 226 else 227 return false; 228 } 229 public boolean validateATM(String ATMid){ 230 if(ATMid.matches("0[1-6]")){ 231 return true; 232 } 233 else 234 return false; 235 } 236 public Account validateCardNum(String cardNum){ 237 CheckCard checkCard=new CheckCard(this.chinaUnionPay); 238 Account account=checkCard.QueryAccount(cardNum); 239 return account; 240 } 241 public boolean validateData(){ 242 int flag = 0; 243 String data[] = datas.split(" "); 244 ArrayList<String> list = new ArrayList<>(); 245 for (int i = 0; i < data.length; i++) { 246 list.add(data[i]); 247 list.remove(""); 248 } 249 Account account = validateCardNum(list.get(0)); 250 CheckCard checkCard = new CheckCard(this.chinaUnionPay); 251 Bank bank = checkCard.QueryBank(list.get(0)); 252 if (account==null) { 253 System.out.println("Sorry,this card does not exist."); 254 return false; 255 } 256 if (list.size() == 4) { 257 flag = 1; 258 } else if (list.size() == 1) { 259 flag = 2; 260 } 261 if (flag == 1) { 262 if (!validateATM(list.get(2))) { 263 System.out.println("Sorry,the Main.ATM's id is wrong."); 264 return false; 265 } 266 if (!validatePassword(list.get(1))) { 267 System.out.println("Sorry,your password is wrong."); 268 return false; 269 } 270 if (!validateGetMoney(Double.parseDouble(list.get(3)), account)) { 271 System.out.println("Sorry,your account balance is insufficient."); 272 return false; 273 } 274 boolean key = true; 275 if (list.get(2).equals("01") || list.get(2).equals("02") || list.get(2).equals("03") || list.get(2).equals("04") && bank.getName().equals("中国建设银行")) { 276 key = true; 277 } else if (list.get(2).equals("05") || list.get(2).equals("06") && bank.getName().equals("中国工商银行")) { 278 key = true; 279 } else { 280 key = false; 281 } 282 if (key == false) { 283 System.out.println("Sorry,cross-bank withdrawal is not supported."); 284 return false; 285 } 286 } 287 return true; 288 } 289 } 290 291 public static class CheckCard { 292 private ChinaUnionPay chinaUnionPay; 293 private String ATMid; 294 public CheckCard() { 295 } 296 297 public CheckCard(ChinaUnionPay chinaUnionPay, String ATMid) { 298 this.chinaUnionPay = chinaUnionPay; 299 this.ATMid = ATMid; 300 } 301 public CheckCard(ChinaUnionPay chinaUnionPay) { 302 this.chinaUnionPay = chinaUnionPay; 303 } 304 305 public String getATMid() { 306 return ATMid; 307 } 308 309 public void setATMid(String ATMid) { 310 this.ATMid = ATMid; 311 } 312 313 public Account QueryAccount(String cardNumber) { 314 Account account = null; 315 out: 316 for (int i = 0; i < chinaUnionPay.getBanks().length; i++) { 317 for (int j = 0; j < chinaUnionPay.getBanks()[i].getUsers().length; j++) { 318 for (int k = 0; k < chinaUnionPay.getBanks()[i].getUsers()[j].getAccounts().length; k++) { 319 for (int l = 0; l < chinaUnionPay.getBanks()[i].getUsers()[j].getAccounts()[k].getCards().length; l++) { 320 if (chinaUnionPay.getBanks()[i].getUsers()[j].getAccounts()[k].getCards()[l].getCardNum().equals(cardNumber)) { 321 account = chinaUnionPay.getBanks()[i].getUsers()[j].getAccounts()[k]; 322 break out; 323 } 324 } 325 } 326 } 327 } 328 return account; 329 // for(int i=0;i<chinaUnionPay.getUsers().length;i++){ 330 // for(int j=0;j<chinaUnionPay.getUsers()[i].getAccounts().length;j++){ 331 // for (int k=0;k<chinaUnionPay.getUsers()[i].getAccounts()[j].getCards().length;k++){ 332 // if(chinaUnionPay.getUsers()[i].getAccounts()[j].getCards()[k].getCardNum().equals(cardNumber)){ 333 // account=chinaUnionPay.getUsers()[i].getAccounts()[j]; 334 // break out; 335 // } 336 // } 337 // } 338 // } 339 // return account; 340 } 341 342 public User QueryUser(String cardNumber) { 343 User user = new User(); 344 out: 345 for (int i = 0; i < chinaUnionPay.getBanks().length; i++) { 346 for (int j = 0; j < chinaUnionPay.getBanks()[i].getUsers().length; j++) { 347 for (int k = 0; k < chinaUnionPay.getBanks()[i].getUsers()[j].getAccounts().length; k++) { 348 for (int l = 0; l < chinaUnionPay.getBanks()[i].getUsers()[j].getAccounts()[k].getCards().length; l++) { 349 if (chinaUnionPay.getBanks()[i].getUsers()[j].getAccounts()[k].getCards()[l].getCardNum().equals(cardNumber)) { 350 user = chinaUnionPay.getBanks()[i].getUsers()[j]; 351 break out; 352 } 353 } 354 } 355 } 356 } 357 // for (int i=0;i<chinaUnionPay.getUsers().length;i++){ 358 // for (int j=0;j<chinaUnionPay.getUsers()[i].getAccounts().length;j++){ 359 // for (int k=0;k<chinaUnionPay.getUsers()[i].getAccounts()[j].getCards().length;k++){ 360 // if(chinaUnionPay.getUsers()[i].getAccounts()[j].getCards()[k].getCardNum().equals(cardNumber)){ 361 // user=chinaUnionPay.getUsers()[i]; 362 // break out; 363 // } 364 // } 365 // } 366 // } 367 return user; 368 } 369 370 public Bank QueryBank(String cardNumber) { 371 Bank bank = new Bank(); 372 out: 373 for (int i = 0; i < chinaUnionPay.getBanks().length; i++) { 374 for (int j = 0; j < chinaUnionPay.getBanks()[i].getUsers().length; j++) { 375 for (int k = 0; k < chinaUnionPay.getBanks()[i].getUsers()[j].getAccounts().length; k++) { 376 for (int l = 0; l < chinaUnionPay.getBanks()[i].getUsers()[j].getAccounts()[k].getCards().length; l++) { 377 if (chinaUnionPay.getBanks()[i].getUsers()[j].getAccounts()[k].getCards()[l].getCardNum().equals(cardNumber)) { 378 bank = chinaUnionPay.getBanks()[i]; 379 break out; 380 } 381 } 382 } 383 } 384 } 385 386 return bank; 387 } 388 } 389 390 public static class Card { 391 private String cardNum;//卡号 392 private String password="88888888"; 393 public Card(){} 394 public Card(String cardNum){ 395 this.cardNum=cardNum; 396 } 397 398 public String getCardNum() { 399 return cardNum; 400 } 401 402 public void setCardNum(String cardNum) { 403 this.cardNum = cardNum; 404 } 405 } 406 407 public static class Bank { 408 private ATM[] atms; 409 private String name; 410 private User[] users; 411 public Bank() { 412 } 413 414 public Bank(String name, ATM[] atms,User[] users) { 415 this.name = name; 416 this.users = users; 417 this.atms = atms; 418 } 419 420 public String getName() { 421 return name; 422 } 423 424 public void setName(String name) { 425 this.name = name; 426 } 427 428 public User[] getUsers() { 429 return users; 430 } 431 432 public void setUsers(User[] users) { 433 this.users = users; 434 } 435 } 436 437 public static class ATM { 438 private String id; 439 440 // 构造函数 441 public ATM(String id) { 442 this.id = id; 443 } 444 public ATM(){} 445 446 public String getId() { 447 return id; 448 } 449 450 public void setId(String id) { 451 this.id = id; 452 } 453 } 454 455 public static class Account { 456 private String accountNum;//账号 457 private double balance=10000.00;//初始余额 458 private Card[] cards; 459 460 // 构造函数 461 public Account(String accountNum, Card[] cards) { 462 this.accountNum = accountNum; 463 this.cards = cards; 464 } 465 public Account(){} 466 467 public double getBalance() { 468 return balance; 469 } 470 471 public Card[] getCards() { 472 return cards; 473 } 474 475 public void setCards(Card[] cards) { 476 this.cards = cards; 477 } 478 479 public String getAccountNum() { 480 return accountNum; 481 } 482 483 public void setAccountNum(String accountNum) { 484 this.accountNum = accountNum; 485 } 486 487 public void setBalance(double balance) { 488 this.balance = balance; 489 } 490 } 491 }
这道题对我来说难度也偏高,需求比较复杂且麻烦。
以下为我的设计类图:
5. ATM机类结构设计(二)
题目说明:https://images.ptausercontent.com/60abe58e-09db-49b0-b9dc-219a33d2c073.pdf
这道题在上一题的基础上增加了跨行的业务处理,我有部分代码是直接延用ATM(一)的代码,但由于我ATM(一)的代码也写得很答辩,花了大量的时间在ATM(一)上仍没写出个什么,导致ATM(二)我也没写出来。
三、踩坑心得
1.日期问题面向对象设计(聚合一)
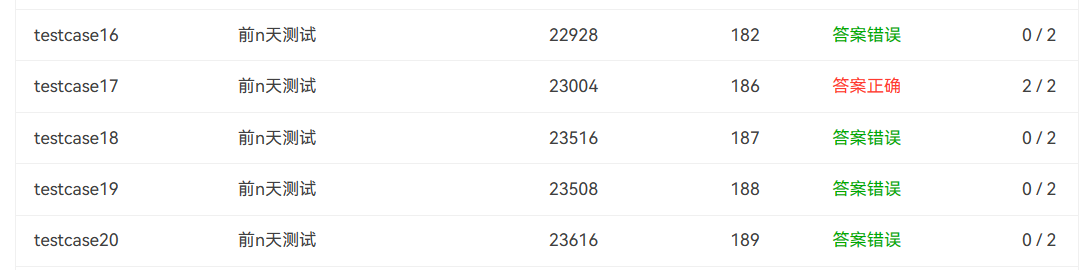
部分方法没有完整地考虑所有可能的情况,getNextNDays() 和 getPreviousNDays() 中对月份和年份的变化的处理可能会出现错误,果然在getPreviousNDays()方法中出错了。
前几次测试点都是前n天测试过不去,一直在小小的修改都过不去,后面直接重写了getPreviousNDays()方法才行。

1 public DateUtil getPreviousNDays(int n) {//求前n天 2 DateUtil prevDate = new DateUtil(this.day.getMonth().getYear().getValue(), 3 this.day.getMonth().getValue(), this.day.getValue()); 4 for (int i = 0; i < n; i++) { 5 prevDate.day.dayReduction(); 6 if (!prevDate.day.validate()) { 7 8 prevDate.day.getMonth().monthReduction(); 9 if (!prevDate.day.getMonth().validate()) { 10 prevDate.day.getMonth().resetMax(); 11 prevDate.day.getMonth().getYear().yearReduction(); 12 } 13 prevDate.day.resetMax(); 14 } 15 } 16 return prevDate; 17 }
2.日期问题面向对象设计(聚合二)
这道题一直在整数型最大值测试点卡住,一开始是运行超时,后面改着改着不会超时了,但是引起了一连串的其它错误,于是我后来干脆直接更换了写法。
这是我之前的写法:

1 public DateUtil getNextNDays(int n) {//求下n天 2 DateUtil nextDate = new DateUtil(this.year.getValue(), 3 this.month.getValue(), this.day.getValue()); 4 for (int i = 0; i < n; i++) { 5 nextDate.day.dayIncreament(); 6 if(nextDate.year.getValue()>2020) { 7 nextDate.month.monthIncrement(); 8 if (!nextDate.month.validate()) { 9 nextDate.month.resetMin(); 10 nextDate.year.yearIncrement(); 11 } 12 nextDate.setDayMin(); 13 } 14 else if (!nextDate.checkInputValidity()) { 15 nextDate.month.monthIncrement(); 16 if (!nextDate.month.validate()) { 17 nextDate.month.resetMin(); 18 nextDate.year.yearIncrement(); 19 } 20 nextDate.setDayMin(); 21 } 22 23 } 24 return nextDate; 25 }
后面改写成:

1 public DateUtil getNextNDays(int n) {//求下n天 2 DateUtil nextDate = new DateUtil(this.year.getValue(), 3 this.month.getValue(), this.day.getValue()); 4 for (int i = 0; i < n; i++) { 5 if(nextDate.year.isLeapYear()) { 6 mon_maxnum[1] = 29; 7 } 8 else { 9 mon_maxnum[1] = 28; 10 } 11 if(nextDate.month.getValue()==12&&nextDate.day.getValue()==31){ 12 nextDate.year.yearIncrement(); 13 nextDate.month.resetMin(); 14 nextDate.setDayMin(); 15 } 16 else if(nextDate.month.getValue()!=12 && nextDate.day.getValue()==mon_maxnum[nextDate.month.getValue()-1]){ 17 nextDate.month.monthIncrement(); 18 nextDate.setDayMin(); 19 } 20 else{ 21 nextDate.day.dayIncreament(); 22 } 23 } 24 return nextDate; 25 }
4&5. ATM机类结构设计
这是第一次提交的提交结果详情,很混乱,我也不明白为什么ATM编号会出错。
第一次提交的代码如下:

1 import java.util.ArrayList; 2 import java.util.Scanner; 3 4 public class Main { 5 6 public static void main(String[] args) { 7 ChinaUnionPay chinaUnionPay=init(); // 初始化数据 8 Scanner scanner = new Scanner(System.in); 9 String line; 10 StringBuilder sb=new StringBuilder(); 11 line=scanner.nextLine(); 12 while (!line.equals("#")) { 13 sb.append(line + "\n"); 14 line = scanner.nextLine(); 15 } 16 Deal deal=new Deal(chinaUnionPay,sb); 17 deal.getDealResult(); 18 19 } 20 21 // 初始化数据 22 private static ChinaUnionPay init() { 23 Card card1=new Card("6217000010041315709"); 24 Card card2=new Card("6217000010041315715"); 25 Card card3=new Card("6217000010041315718"); 26 Card card4=new Card("6217000010051320007"); 27 Card card5=new Card("6222081502001312389"); 28 Card card6=new Card("6222081502001312390"); 29 Card card7=new Card("6222081502001312399"); 30 Card card8=new Card("6222081502001312400"); 31 Card card9=new Card("6222081502051320785"); 32 Card card10=new Card("6222081502051320786"); 33 Card[] cards1={card1,card2}; 34 Card[] cards2={card3}; 35 Card[] cards3={card4}; 36 Card[] cards4={card5}; 37 Card[] cards5={card6}; 38 Card[] cards6={card7,card8}; 39 Card[] cards7={card9}; 40 Card[] cards8={card10}; 41 42 Account account1=new Account("3217000010041315709",cards1); 43 Account account2=new Account("3217000010041315715",cards2); 44 Account account3=new Account("3217000010051320007",cards3); 45 Account account4=new Account("3222081502001312389",cards4); 46 Account account5=new Account("3222081502001312390",cards5); 47 Account account6=new Account("3222081502001312399",cards6); 48 Account account7=new Account("3222081502051320785",cards7); 49 Account account8=new Account("3222081502051320786",cards8); 50 Account[] accounts1={account1,account2}; 51 Account[] accounts2={account3}; 52 Account[] accounts3={account4,account5,account6}; 53 Account[] accounts4={account7,account8}; 54 55 ATM atm1=new ATM("01"); 56 ATM atm2=new ATM("02"); 57 ATM atm3=new ATM("03"); 58 ATM atm4=new ATM("04"); 59 ATM atm5=new ATM("05"); 60 ATM atm6=new ATM("06"); 61 ATM[] atms1={atm1,atm2,atm3,atm4}; 62 ATM[] atms2={atm5,atm6}; 63 64 Bank bank1=new Bank("中国建设银行",atms1); 65 Bank bank2=new Bank("中国工商银行",atms2); 66 Bank[] banks={bank1,bank2}; 67 68 User user1=new User("杨过",accounts1,bank1); 69 User user2=new User("郭靖",accounts2,bank1); 70 User user3=new User("张无忌",accounts3,bank2); 71 User user4=new User("韦小宝",accounts4,bank2); 72 User[] users={user1,user2,user3,user4}; 73 74 ChinaUnionPay chinaUnionPay=new ChinaUnionPay(banks,users); 75 return chinaUnionPay; 76 } 77 78 public static class User { 79 private String userName; 80 private Account[] accounts; 81 private Bank bank; 82 public User(){} 83 public User(String userName,Account[] accounts,Bank bank){ 84 this.userName=userName; 85 this.accounts=accounts; 86 this.bank = bank; 87 } 88 89 public Bank getBank() { 90 return bank; 91 } 92 93 public void setBank(Bank bank) { 94 this.bank = bank; 95 } 96 97 public Account[] getAccounts() { 98 return accounts; 99 } 100 101 public void setAccounts(Account[] accounts) { 102 this.accounts = accounts; 103 } 104 105 public String getUserName() { 106 return userName; 107 } 108 109 public void setUserName(String userName) { 110 this.userName = userName; 111 } 112 } 113 114 public static class Deal { 115 private ChinaUnionPay chinaUnionPay; 116 private StringBuilder sb; 117 public Deal(){} 118 public Deal(ChinaUnionPay chinaUnionPay,StringBuilder sb){ 119 this.chinaUnionPay=chinaUnionPay; 120 this.sb=sb; 121 } 122 123 public StringBuilder getSb() { 124 return sb; 125 } 126 127 public void setSb(StringBuilder sb) { 128 this.sb = sb; 129 } 130 131 public ChinaUnionPay getChinaUnionPay() { 132 return chinaUnionPay; 133 } 134 135 public void setChinaUnionPay(ChinaUnionPay chinaUnionPay) { 136 this.chinaUnionPay = chinaUnionPay; 137 } 138 public void getDealResult(){ 139 String datas= sb.toString(); 140 String[] data=datas.split("\n"); 141 for(int i=0;i<data.length;i++){ 142 CheckData checkData=new CheckData(data[i],this.chinaUnionPay); 143 if(checkData.validateData()){ 144 String part[] =data[i].split(" "); 145 ArrayList<String> arrayList=new ArrayList<>(); 146 for (int j=0;j< part.length;j++){ 147 arrayList.add(part[j]); 148 arrayList.remove(""); 149 } 150 if(arrayList.size()==4){ 151 CheckCard checkCard1=new CheckCard(this.chinaUnionPay,arrayList.get(2)); 152 changeBalance(checkCard1,arrayList.get(0), Double.parseDouble(arrayList.get(3))); 153 } 154 else if(arrayList.size()==1){ 155 CheckCard checkCard2=new CheckCard(this.chinaUnionPay); 156 Account account=checkCard2.QueryAccount(arrayList.get(0)); 157 System.out.print("¥"); 158 System.out.printf("%.2f\n",account.getBalance()); 159 } 160 } 161 } 162 } 163 public void changeBalance(CheckCard checkCard,String cardNumber, double money) { //存钱或取钱 164 Account account = checkCard.QueryAccount(cardNumber); 165 User user = checkCard.QueryUser(cardNumber); 166 Bank bank = checkCard.QueryBank(cardNumber); 167 account.setBalance(account.getBalance() - money); 168 if (money > 0) { 169 System.out.println(user.getUserName() + "在" + bank.getName() + "的" + checkCard.getATMid() + "号ATM机上取款¥" + String.format("%.2f", money) + "\n" + "当前余额为¥" + String.format("%.2f", account.getBalance())); 170 } else { 171 System.out.println(user.getUserName() + "在" + bank.getName() + "的" + checkCard.getATMid() + "号ATM机上存款¥" + String.format("%.2f", -money) + "\n" + "当前余额为¥" + String.format("%.2f", account.getBalance())); 172 } 173 } 174 } 175 176 public static class ChinaUnionPay { 177 private Bank[] banks; 178 private User[] users; 179 public ChinaUnionPay(){} 180 public ChinaUnionPay(Bank[] banks, User[] users){ 181 this.banks=banks; 182 this.users = users; 183 } 184 185 public Bank[] getBanks() { 186 return banks; 187 } 188 189 public void setBanks(Bank[] banks) { 190 this.banks = banks; 191 } 192 193 public User[] getUsers() { 194 return users; 195 } 196 197 public void setUsers(User[] users) { 198 this.users = users; 199 } 200 } 201 202 public static class CheckData { 203 private String datas; 204 private ChinaUnionPay chinaUnionPay; 205 206 public CheckData() { 207 } 208 public CheckData(String data, ChinaUnionPay chinaUnionPay){ 209 this.chinaUnionPay = chinaUnionPay; 210 this.datas = data; 211 } 212 213 public ChinaUnionPay getChinaUnionPay() { 214 return chinaUnionPay; 215 } 216 217 public void setChinaUnionPay(ChinaUnionPay chinaUnionPay) { 218 this.chinaUnionPay = chinaUnionPay; 219 } 220 221 public String getData() { 222 return datas; 223 } 224 225 public void setData(String datas) { 226 this.datas = datas; 227 } 228 public boolean validateGetMoney(double withdrawMoney, Account account) { 229 if (withdrawMoney > account.getBalance()) { 230 return false; 231 } 232 else 233 return true; 234 } 235 public boolean validatePassword(String password){ 236 if(password.matches("88888888")){ 237 return true; 238 } 239 else 240 return false; 241 } 242 public boolean validateATM(String ATMid){ 243 if(ATMid.matches("0[1-6]")){ 244 return true; 245 } 246 else 247 return false; 248 } 249 public Account validateCardNum(String cardNum){ 250 CheckCard checkCard=new CheckCard(this.chinaUnionPay); 251 Account account=checkCard.QueryAccount(cardNum); 252 return account; 253 } 254 public boolean validateData(){ 255 int flag = 0; 256 String data[] = datas.split(" "); 257 ArrayList<String> list = new ArrayList<>(); 258 for (int i = 0; i < data.length; i++) { 259 list.add(data[i]); 260 list.remove(""); 261 } 262 Account account = validateCardNum(list.get(0)); 263 CheckCard checkCard = new CheckCard(this.chinaUnionPay); 264 Bank bank = checkCard.QueryBank(list.get(0)); 265 if (account == null) { 266 System.out.println("Sorry,this card does not exist."); 267 return false; 268 } 269 if (list.size() == 4) { 270 flag = 1; 271 } else if (list.size() == 1) { 272 flag = 2; 273 } 274 if (flag == 1) { 275 if (!validateATM(list.get(2))) { 276 System.out.println("Sorry,the Main.ATM's id is wrong."); 277 return false; 278 } 279 if (!validatePassword(list.get(1))) { 280 System.out.println("Sorry,your password is wrong."); 281 return false; 282 } 283 if (!validateGetMoney(Double.parseDouble(list.get(3)), account)) { 284 System.out.println("Sorry,your account balance is insufficient."); 285 return false; 286 } 287 boolean key = true; 288 if (list.get(2).equals("01") || list.get(2).equals("02") || list.get(2).equals("03") || list.get(2).equals("04") && bank.getName().equals("中国建设银行")) { 289 key = true; 290 } else if (list.get(2).equals("05") || list.get(2).equals("06") && bank.getName().equals("中国工商银行")) { 291 key = true; 292 } else { 293 key = false; 294 } 295 if (key == false) { 296 System.out.println("Sorry,cross-bank withdrawal is not supported."); 297 return false; 298 } 299 } 300 return true; 301 } 302 } 303 304 public static class CheckCard { 305 private ChinaUnionPay chinaUnionPay; 306 private String ATMid; 307 public CheckCard() { 308 } 309 310 public CheckCard(ChinaUnionPay chinaUnionPay, String ATMid) { 311 this.chinaUnionPay = chinaUnionPay; 312 this.ATMid = ATMid; 313 } 314 public CheckCard(ChinaUnionPay chinaUnionPay) { 315 this.chinaUnionPay = chinaUnionPay; 316 } 317 318 public String getATMid() { 319 return ATMid; 320 } 321 322 public void setATMid(String ATMid) { 323 this.ATMid = ATMid; 324 } 325 326 public Account QueryAccount(String cardNumber) { 327 Account account = null; 328 out: 329 // for (int i = 0; i < chinaUnionPay.getBanks().length; i++) { 330 // for (int j = 0; j < chinaUnionPay.getBanks()[i].getUsers().length; j++) { 331 // for (int k = 0; k < chinaUnionPay.getBanks()[i].getUsers()[j].getAccounts().length; k++) { 332 // for (int l = 0; l < chinaUnionPay.getBanks()[i].getUsers()[j].getAccounts()[k].getCards().length; l++) { 333 // if (chinaUnionPay.getBanks()[i].getUsers()[j].getAccounts()[k].getCards()[l].getCardNumber().equals(cardNumber)) { 334 // account = chinaUnionPay.getBanks()[i].getUsers()[j].getAccounts()[k]; 335 // break out; 336 // } 337 // } 338 // } 339 // } 340 // } 341 // return account; 342 for(int i=0;i<chinaUnionPay.getUsers().length;i++){ 343 for(int j=0;j<chinaUnionPay.getUsers()[i].getAccounts().length;j++){ 344 for (int k=0;k<chinaUnionPay.getUsers()[i].getAccounts()[j].getCards().length;k++){ 345 if(chinaUnionPay.getUsers()[i].getAccounts()[j].getCards()[k].getCardNum().equals(cardNumber)){ 346 account=chinaUnionPay.getUsers()[i].getAccounts()[j]; 347 break out; 348 } 349 } 350 } 351 } 352 return account; 353 } 354 355 public User QueryUser(String cardNumber) { 356 User user = new User(); 357 out: 358 // for (int i = 0; i < chinaUnionPay.getBanks().length; i++) { 359 // for (int j = 0; j < chinaUnionPay.getBanks()[i].getUsers().length; j++) { 360 // for (int k = 0; k < chinaUnionPay.getBanks()[i].getUsers()[j].getAccounts().length; k++) { 361 // for (int l = 0; l < chinaUnionPay.getBanks()[i].getUsers()[j].getAccounts()[k].getCards().length; l++) { 362 // if (chinaUnionPay.getBanks()[i].getUsers()[j].getAccounts()[k].getCards()[l].getCardNumber().equals(cardNumber)) { 363 // user = chinaUnionPay.getBanks()[i].getUsers()[j]; 364 // break out; 365 // } 366 // } 367 // } 368 // } 369 // } 370 for (int i=0;i<chinaUnionPay.getUsers().length;i++){ 371 for (int j=0;j<chinaUnionPay.getUsers()[i].getAccounts().length;j++){ 372 for (int k=0;k<chinaUnionPay.getUsers()[i].getAccounts()[j].getCards().length;k++){ 373 if(chinaUnionPay.getUsers()[i].getAccounts()[j].getCards()[k].getCardNum().equals(cardNumber)){ 374 user=chinaUnionPay.getUsers()[i]; 375 break out; 376 } 377 } 378 } 379 } 380 return user; 381 } 382 383 public Bank QueryBank(String cardNumber) { 384 Bank bank = new Bank(); 385 out: 386 for (int i = 0; i < chinaUnionPay.getBanks().length; i++) { 387 for (int j = 0; j < chinaUnionPay.getUsers().length; j++) { 388 for (int k = 0; k < chinaUnionPay.getUsers()[j].getAccounts().length; k++) { 389 for (int l = 0; l < chinaUnionPay.getUsers()[j].getAccounts()[k].getCards().length; l++) { 390 if (chinaUnionPay.getUsers()[j].getAccounts()[k].getCards()[l].getCardNum().equals(cardNumber)) { 391 bank = chinaUnionPay.getBanks()[i]; 392 break out; 393 } 394 } 395 } 396 } 397 } 398 399 return bank; 400 } 401 } 402 403 public static class Card { 404 private String cardNum;//卡号 405 private String password="88888888"; 406 public Card(){} 407 public Card(String cardNum){ 408 this.cardNum=cardNum; 409 } 410 411 public String getCardNum() { 412 return cardNum; 413 } 414 415 public void setCardNum(String cardNum) { 416 this.cardNum = cardNum; 417 } 418 } 419 420 public static class Bank { 421 private String name; 422 private ATM[] ATMs; 423 424 // 构造函数 425 public Bank(String name,ATM[] ATMs) { 426 this.name = name; 427 this.ATMs = ATMs; 428 } 429 public Bank(){} 430 431 // 获取银行名称 432 public String getName() { 433 return name; 434 } 435 436 public void setName(String name) { 437 this.name = name; 438 } 439 440 public ATM[] getATMs() { 441 return ATMs; 442 } 443 444 public void setATMs(ATM[] ATMs) { 445 this.ATMs = ATMs; 446 } 447 } 448 449 public static class ATM { 450 private String id; 451 452 // 构造函数 453 public ATM(String id) { 454 this.id = id; 455 } 456 public ATM(){} 457 458 public String getId() { 459 return id; 460 } 461 462 public void setId(String id) { 463 this.id = id; 464 } 465 } 466 467 public static class Account { 468 private String accountNum;//账号 469 private double balance=10000.00;//初始余额 470 private Card[] cards; 471 472 // 构造函数 473 public Account(String accountNum, Card[] cards) { 474 this.accountNum = accountNum; 475 this.cards = cards; 476 } 477 public Account(){} 478 479 public double getBalance() { 480 return balance; 481 } 482 483 public Card[] getCards() { 484 return cards; 485 } 486 487 public void setCards(Card[] cards) { 488 this.cards = cards; 489 } 490 491 public String getAccountNum() { 492 return accountNum; 493 } 494 495 public void setAccountNum(String accountNum) { 496 this.accountNum = accountNum; 497 } 498 499 public void setBalance(double balance) { 500 this.balance = balance; 501 } 502 } 503 }
后面又重新写过,提交结果详情如下:
代码如下:

1 import java.util.ArrayList; 2 import java.util.Scanner; 3 4 public class Main { 5 6 public static void main(String[] args) { 7 ChinaUnionPay chinaUnionPay=init(); // 初始化数据 8 Scanner scanner = new Scanner(System.in); 9 String line; 10 StringBuilder sb=new StringBuilder(); 11 line=scanner.nextLine(); 12 while (!line.equals("#")) { 13 sb.append(line + "\n"); 14 line = scanner.nextLine(); 15 } 16 Deal deal=new Deal(chinaUnionPay,sb); 17 deal.getDealResult(); 18 19 } 20 21 // 初始化数据 22 private static ChinaUnionPay init() { 23 Card card1=new Card("6217000010041315709"); 24 Card card2=new Card("6217000010041315715"); 25 Card card3=new Card("6217000010041315718"); 26 Card card4=new Card("6217000010051320007"); 27 Card card5=new Card("6222081502001312389"); 28 Card card6=new Card("6222081502001312390"); 29 Card card7=new Card("6222081502001312399"); 30 Card card8=new Card("6222081502001312400"); 31 Card card9=new Card("6222081502051320785"); 32 Card card10=new Card("6222081502051320786"); 33 Card[] cards1={card1,card2}; 34 Card[] cards2={card3}; 35 Card[] cards3={card4}; 36 Card[] cards4={card5}; 37 Card[] cards5={card6}; 38 Card[] cards6={card7,card8}; 39 Card[] cards7={card9}; 40 Card[] cards8={card10}; 41 42 Account account1=new Account("3217000010041315709",cards1); 43 Account account2=new Account("3217000010041315715",cards2); 44 Account account3=new Account("3217000010051320007",cards3); 45 Account account4=new Account("3222081502001312389",cards4); 46 Account account5=new Account("3222081502001312390",cards5); 47 Account account6=new Account("3222081502001312399",cards6); 48 Account account7=new Account("3222081502051320785",cards7); 49 Account account8=new Account("3222081502051320786",cards8); 50 Account[] accounts1={account1,account2}; 51 Account[] accounts2={account3}; 52 Account[] accounts3={account4,account5,account6}; 53 Account[] accounts4={account7,account8}; 54 55 ATM atm1=new ATM("01"); 56 ATM atm2=new ATM("02"); 57 ATM atm3=new ATM("03"); 58 ATM atm4=new ATM("04"); 59 ATM atm5=new ATM("05"); 60 ATM atm6=new ATM("06"); 61 ATM[] atms1={atm1,atm2,atm3,atm4}; 62 ATM[] atms2={atm5,atm6}; 63 64 65 User user1=new User("杨过",accounts1); 66 User user2=new User("郭靖",accounts2); 67 User user3=new User("张无忌",accounts3); 68 User user4=new User("韦小宝",accounts4); 69 User[] users1={user1,user2}; 70 User[] users2={user3,user4}; 71 72 Bank bank1=new Bank("中国建设银行",atms1,users1); 73 Bank bank2=new Bank("中国工商银行",atms2,users2); 74 Bank[] banks={bank1,bank2}; 75 76 77 ChinaUnionPay chinaUnionPay=new ChinaUnionPay(banks); 78 return chinaUnionPay; 79 } 80 81 public static class User { 82 private String userName; 83 private Account[] accounts; 84 public User(){} 85 public User(String userName,Account[] accounts){ 86 this.userName=userName; 87 this.accounts=accounts; 88 } 89 90 public Account[] getAccounts() { 91 return accounts; 92 } 93 94 public void setAccounts(Account[] accounts) { 95 this.accounts = accounts; 96 } 97 98 public String getUserName() { 99 return userName; 100 } 101 102 public void setUserName(String userName) { 103 this.userName = userName; 104 } 105 } 106 107 public static class Deal { 108 private ChinaUnionPay chinaUnionPay; 109 private StringBuilder sb; 110 public Deal(){} 111 public Deal(ChinaUnionPay chinaUnionPay,StringBuilder sb){ 112 this.chinaUnionPay=chinaUnionPay; 113 this.sb=sb; 114 } 115 116 public StringBuilder getSb() { 117 return sb; 118 } 119 120 public void setSb(StringBuilder sb) { 121 this.sb = sb; 122 } 123 124 public ChinaUnionPay getChinaUnionPay() { 125 return chinaUnionPay; 126 } 127 128 public void setChinaUnionPay(ChinaUnionPay chinaUnionPay) { 129 this.chinaUnionPay = chinaUnionPay; 130 } 131 public void getDealResult(){ 132 String datas= sb.toString(); 133 String[] data=datas.split("\n"); 134 for(int i=0;i<data.length;i++){ 135 CheckData checkData=new CheckData(data[i],this.chinaUnionPay); 136 if(checkData.validateData()){ 137 String part[] =data[i].split(" "); 138 ArrayList<String> arrayList=new ArrayList<>(); 139 for (int j=0;j< part.length;j++){ 140 arrayList.add(part[j]); 141 arrayList.remove(""); 142 } 143 if(arrayList.size()==4){ 144 CheckCard checkCard1=new CheckCard(this.chinaUnionPay,arrayList.get(2)); 145 changeBalance(checkCard1,arrayList.get(0), Double.parseDouble(arrayList.get(3))); 146 } 147 else if(arrayList.size()==1){ 148 CheckCard checkCard2=new CheckCard(this.chinaUnionPay); 149 Account account=checkCard2.QueryAccount(arrayList.get(0)); 150 System.out.print("¥"); 151 System.out.printf("%.2f\n",account.getBalance()); 152 } 153 } 154 else if(!checkData.validateData()){ 155 return; 156 } 157 } 158 } 159 public void changeBalance(CheckCard checkCard,String cardNumber, double money) { //存钱或取钱 160 Account account = checkCard.QueryAccount(cardNumber); 161 User user = checkCard.QueryUser(cardNumber); 162 Bank bank = checkCard.QueryBank(cardNumber); 163 account.setBalance(account.getBalance() - money); 164 if (money > 0) { 165 System.out.println(user.getUserName() + "在" + bank.getName() + "的" + checkCard.getATMid() + "号ATM机上取款¥" + String.format("%.2f", money) + "\n" + "当前余额为¥" + String.format("%.2f", account.getBalance())); 166 } else { 167 System.out.println(user.getUserName() + "在" + bank.getName() + "的" + checkCard.getATMid() + "号ATM机上存款¥" + String.format("%.2f", -money) + "\n" + "当前余额为¥" + String.format("%.2f", account.getBalance())); 168 } 169 } 170 } 171 172 public static class ChinaUnionPay { 173 private Bank[] banks; 174 public ChinaUnionPay(){} 175 public ChinaUnionPay(Bank[] banks){ 176 this.banks=banks; 177 } 178 179 public Bank[] getBanks() { 180 return banks; 181 } 182 183 public void setBanks(Bank[] banks) { 184 this.banks = banks; 185 } 186 187 } 188 189 public static class CheckData { 190 private String datas; 191 private ChinaUnionPay chinaUnionPay; 192 193 public CheckData() { 194 } 195 public CheckData(String data, ChinaUnionPay chinaUnionPay){ 196 this.chinaUnionPay = chinaUnionPay; 197 this.datas = data; 198 } 199 200 public ChinaUnionPay getChinaUnionPay() { 201 return chinaUnionPay; 202 } 203 204 public void setChinaUnionPay(ChinaUnionPay chinaUnionPay) { 205 this.chinaUnionPay = chinaUnionPay; 206 } 207 208 public String getData() { 209 return datas; 210 } 211 212 public void setData(String datas) { 213 this.datas = datas; 214 } 215 public boolean validateGetMoney(double withdrawMoney, Account account) { 216 if (withdrawMoney > account.getBalance()) { 217 return false; 218 } 219 else 220 return true; 221 } 222 public boolean validatePassword(String password){ 223 if(password.matches("88888888")){ 224 return true; 225 } 226 else 227 return false; 228 } 229 public boolean validateATM(String ATMid){ 230 if(ATMid.matches("0[1-6]")){ 231 return true; 232 } 233 else 234 return false; 235 } 236 public Account validateCardNum(String cardNum){ 237 CheckCard checkCard=new CheckCard(this.chinaUnionPay); 238 Account account=checkCard.QueryAccount(cardNum); 239 return account; 240 } 241 public boolean validateData(){ 242 int flag = 0; 243 String data[] = datas.split(" "); 244 ArrayList<String> list = new ArrayList<>(); 245 for (int i = 0; i < data.length; i++) { 246 list.add(data[i]); 247 list.remove(""); 248 } 249 Account account = validateCardNum(list.get(0)); 250 CheckCard checkCard = new CheckCard(this.chinaUnionPay); 251 Bank bank = checkCard.QueryBank(list.get(0)); 252 if (account==null) { 253 System.out.println("Sorry,this card does not exist."); 254 return false; 255 } 256 if (list.size() == 4) { 257 flag = 1; 258 } else if (list.size() == 1) { 259 flag = 2; 260 } 261 if (flag == 1) { 262 if (!validateATM(list.get(2))) { 263 System.out.println("Sorry,the Main.ATM's id is wrong."); 264 return false; 265 } 266 if (!validatePassword(list.get(1))) { 267 System.out.println("Sorry,your password is wrong."); 268 return false; 269 } 270 if (!validateGetMoney(Double.parseDouble(list.get(3)), account)) { 271 System.out.println("Sorry,your account balance is insufficient."); 272 return false; 273 } 274 boolean key = true; 275 if (list.get(2).equals("01") || list.get(2).equals("02") || list.get(2).equals("03") || list.get(2).equals("04") && bank.getName().equals("中国建设银行")) { 276 key = true; 277 } else if (list.get(2).equals("05") || list.get(2).equals("06") && bank.getName().equals("中国工商银行")) { 278 key = true; 279 } else { 280 key = false; 281 } 282 if (key == false) { 283 System.out.println("Sorry,cross-bank withdrawal is not supported."); 284 return false; 285 } 286 } 287 return true; 288 } 289 } 290 291 public static class CheckCard { 292 private ChinaUnionPay chinaUnionPay; 293 private String ATMid; 294 public CheckCard() { 295 } 296 297 public CheckCard(ChinaUnionPay chinaUnionPay, String ATMid) { 298 this.chinaUnionPay = chinaUnionPay; 299 this.ATMid = ATMid; 300 } 301 public CheckCard(ChinaUnionPay chinaUnionPay) { 302 this.chinaUnionPay = chinaUnionPay; 303 } 304 305 public String getATMid() { 306 return ATMid; 307 } 308 309 public void setATMid(String ATMid) { 310 this.ATMid = ATMid; 311 } 312 313 public Account QueryAccount(String cardNumber) { 314 Account account = null; 315 out: 316 for (int i = 0; i < chinaUnionPay.getBanks().length; i++) { 317 for (int j = 0; j < chinaUnionPay.getBanks()[i].getUsers().length; j++) { 318 for (int k = 0; k < chinaUnionPay.getBanks()[i].getUsers()[j].getAccounts().length; k++) { 319 for (int l = 0; l < chinaUnionPay.getBanks()[i].getUsers()[j].getAccounts()[k].getCards().length; l++) { 320 if (chinaUnionPay.getBanks()[i].getUsers()[j].getAccounts()[k].getCards()[l].getCardNum().equals(cardNumber)) { 321 account = chinaUnionPay.getBanks()[i].getUsers()[j].getAccounts()[k]; 322 break out; 323 } 324 } 325 } 326 } 327 } 328 return account; 329 // for(int i=0;i<chinaUnionPay.getUsers().length;i++){ 330 // for(int j=0;j<chinaUnionPay.getUsers()[i].getAccounts().length;j++){ 331 // for (int k=0;k<chinaUnionPay.getUsers()[i].getAccounts()[j].getCards().length;k++){ 332 // if(chinaUnionPay.getUsers()[i].getAccounts()[j].getCards()[k].getCardNum().equals(cardNumber)){ 333 // account=chinaUnionPay.getUsers()[i].getAccounts()[j]; 334 // break out; 335 // } 336 // } 337 // } 338 // } 339 // return account; 340 } 341 342 public User QueryUser(String cardNumber) { 343 User user = new User(); 344 out: 345 for (int i = 0; i < chinaUnionPay.getBanks().length; i++) { 346 for (int j = 0; j < chinaUnionPay.getBanks()[i].getUsers().length; j++) { 347 for (int k = 0; k < chinaUnionPay.getBanks()[i].getUsers()[j].getAccounts().length; k++) { 348 for (int l = 0; l < chinaUnionPay.getBanks()[i].getUsers()[j].getAccounts()[k].getCards().length; l++) { 349 if (chinaUnionPay.getBanks()[i].getUsers()[j].getAccounts()[k].getCards()[l].getCardNum().equals(cardNumber)) { 350 user = chinaUnionPay.getBanks()[i].getUsers()[j]; 351 break out; 352 } 353 } 354 } 355 } 356 } 357 // for (int i=0;i<chinaUnionPay.getUsers().length;i++){ 358 // for (int j=0;j<chinaUnionPay.getUsers()[i].getAccounts().length;j++){ 359 // for (int k=0;k<chinaUnionPay.getUsers()[i].getAccounts()[j].getCards().length;k++){ 360 // if(chinaUnionPay.getUsers()[i].getAccounts()[j].getCards()[k].getCardNum().equals(cardNumber)){ 361 // user=chinaUnionPay.getUsers()[i]; 362 // break out; 363 // } 364 // } 365 // } 366 // } 367 return user; 368 } 369 370 public Bank QueryBank(String cardNumber) { 371 Bank bank = new Bank(); 372 out: 373 for (int i = 0; i < chinaUnionPay.getBanks().length; i++) { 374 for (int j = 0; j < chinaUnionPay.getBanks()[i].getUsers().length; j++) { 375 for (int k = 0; k < chinaUnionPay.getBanks()[i].getUsers()[j].getAccounts().length; k++) { 376 for (int l = 0; l < chinaUnionPay.getBanks()[i].getUsers()[j].getAccounts()[k].getCards().length; l++) { 377 if (chinaUnionPay.getBanks()[i].getUsers()[j].getAccounts()[k].getCards()[l].getCardNum().equals(cardNumber)) { 378 bank = chinaUnionPay.getBanks()[i]; 379 break out; 380 } 381 } 382 } 383 } 384 } 385 386 return bank; 387 } 388 } 389 390 public static class Card { 391 private String cardNum;//卡号 392 private String password="88888888"; 393 public Card(){} 394 public Card(String cardNum){ 395 this.cardNum=cardNum; 396 } 397 398 public String getCardNum() { 399 return cardNum; 400 } 401 402 public void setCardNum(String cardNum) { 403 this.cardNum = cardNum; 404 } 405 } 406 407 public static class Bank { 408 private ATM[] atms; 409 private String name; 410 private User[] users; 411 public Bank() { 412 } 413 414 public Bank(String name, ATM[] atms,User[] users) { 415 this.name = name; 416 this.users = users; 417 this.atms = atms; 418 } 419 420 public String getName() { 421 return name; 422 } 423 424 public void setName(String name) { 425 this.name = name; 426 } 427 428 public User[] getUsers() { 429 return users; 430 } 431 432 public void setUsers(User[] users) { 433 this.users = users; 434 } 435 } 436 437 public static class ATM { 438 private String id; 439 440 // 构造函数 441 public ATM(String id) { 442 this.id = id; 443 } 444 public ATM(){} 445 446 public String getId() { 447 return id; 448 } 449 450 public void setId(String id) { 451 this.id = id; 452 } 453 } 454 455 public static class Account { 456 private String accountNum;//账号 457 private double balance=10000.00;//初始余额 458 private Card[] cards; 459 460 // 构造函数 461 public Account(String accountNum, Card[] cards) { 462 this.accountNum = accountNum; 463 this.cards = cards; 464 } 465 public Account(){} 466 467 public double getBalance() { 468 return balance; 469 } 470 471 public Card[] getCards() { 472 return cards; 473 } 474 475 public void setCards(Card[] cards) { 476 this.cards = cards; 477 } 478 479 public String getAccountNum() { 480 return accountNum; 481 } 482 483 public void setAccountNum(String accountNum) { 484 this.accountNum = accountNum; 485 } 486 487 public void setBalance(double balance) { 488 this.balance = balance; 489 } 490 } 491 }
这两道ATM的题目我写的很糟糕,可扩展性非常不好。如果想要增加更多的功能或者修改现有功能,就需要对多个类进行修改,耦合度很高。
还有就是,对异常的处理还是不够完善,比如,当查询的账户或银行卡不存在时,应该抛出异常或者返回错误信息。
四、改进建议
1.日期问题中代码中年、月、日三个类之间的关联较强,且存在大量的获取值和设置值的方法,容易造成代码冗余和重复。
设计代码应该时刻考虑耦合性和可扩展性。
2.代码中缺少注释说明,可读性不够好。
3.部分方法没有完整地考虑所有可能的情况,例如 getNextNDays() 和 getPreviousNDays() 中对月份和年份的变化的处理可能会出现错误。
4.缺少对多线程并发访问的支持。在并发访问时,可能会发生多个线程同时修改同一个账户的余额,这时需要保证数据的一致性和正确性。
5.变量和方法命名不够明确、规范。一些变量和方法的命名可能不太清晰,不够规范,影响代码的可读性和维护性。